Developing a custom WordPress theme allows you to create a unique design tailored specifically to your website’s needs. This process involves several steps, from setting up a local development environment to testing and debugging your theme. Here’s a comprehensive guide on how to create your own custom WordPress theme.
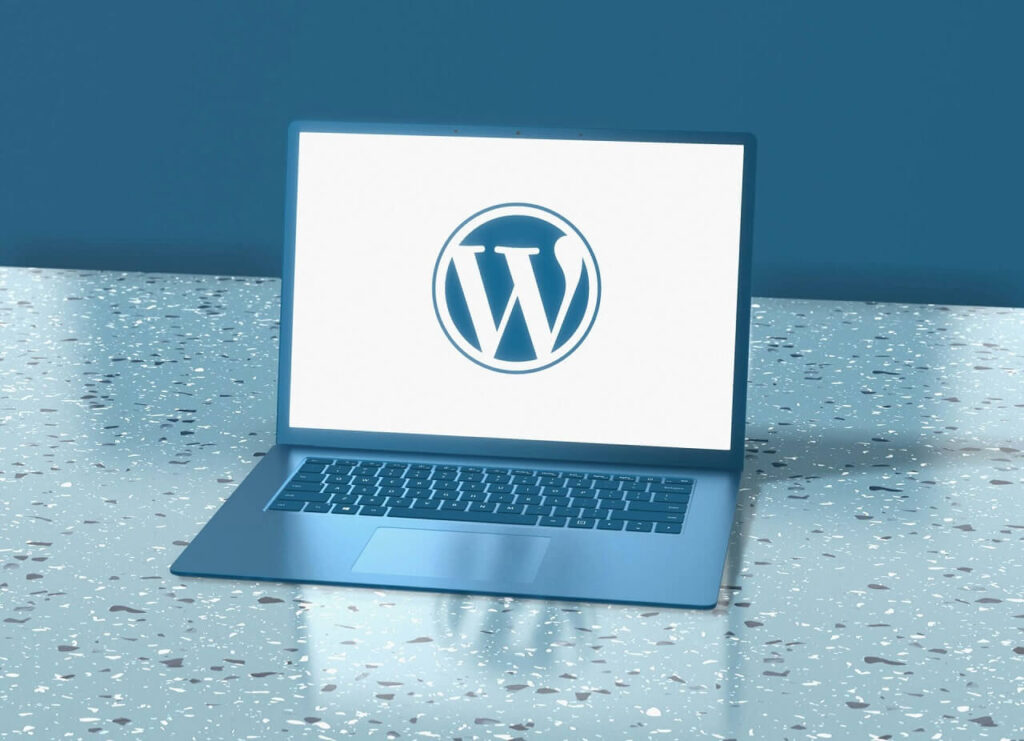
Table of Contents
Setting up a local development environment
The first step in creating a custom WordPress theme is setting up a local development environment. This allows you to work on your theme without affecting your live website. Tools like MAMP, XAMPP, or Local by Flywheel are popular choices for setting up a local server environment. These tools provide a web server, database server, and PHP, which are essential for running WordPress locally.
After setting up your local server, download and install WordPress. You can do this by downloading the latest version from WordPress.org and extracting it to your local server’s root directory. Create a new database for your WordPress installation using phpMyAdmin or a similar tool. Run the WordPress installation script by navigating to your local server’s URL in your web browser and following the on-screen instructions.
With WordPress installed locally, you can now create a new theme directory within the wp-content/themes
folder. This is where all your theme files will reside. Give your theme a unique name to avoid conflicts with existing themes.
Understanding the WordPress theme hierarchy
Before diving into coding, it’s essential to understand the WordPress theme hierarchy. The theme hierarchy dictates how WordPress selects which template files to use for different types of pages. The hierarchy follows a specific order, allowing WordPress to fall back to more general templates if specific ones are not found.
The index.php
file is the most generic template and serves as a fallback for all other templates. More specific templates include single.php
for individual posts, page.php
for static pages, and archive.php
for category or date archives. The header.php
and footer.php
files are used to include common header and footer sections across all templates.
Understanding this hierarchy is crucial for structuring your theme correctly. By creating the appropriate template files, you ensure that WordPress can display your content accurately and consistently. This foundational knowledge will guide you as you build out the various components of your theme.
Creating the basic theme files
With your theme directory set up, it’s time to create the basic theme files. At a minimum, a WordPress theme requires an index.php
file and a style.css
file. The style.css
file should include a theme header comment with details about your theme, such as its name, description, version, and author.
Next, create the index.php
file, which will serve as the primary template for your theme. This file should contain basic HTML structure and calls to WordPress functions like get_header()
and get_footer()
to include the header and footer sections. You can also create additional template files, such as header.php
, footer.php
, single.php
, and page.php
, to handle different types of content.
To add more functionality, create a functions.php
file. This file is where you can add custom PHP code to extend your theme’s capabilities. For example, you can enqueue styles and scripts, register widget areas, and add theme support for features like custom logos and post thumbnails.
Adding custom CSS
Custom CSS is essential for styling your theme to match your design vision. In your style.css
file, you can write the CSS rules that define the appearance of your website. This includes setting fonts, colors, layouts, and responsive design principles to ensure your site looks great on all devices.
To organize your CSS, consider using a modular approach. Break your CSS into multiple files, each handling a specific aspect of your design, such as typography, layout, and components. Use the @import
rule in your style.css
file to include these modular CSS files. This approach makes your CSS more manageable and easier to maintain.
For more advanced styling, you might want to use a CSS preprocessor like Sass. Sass allows you to use variables, nested rules, and mixins, which can make your CSS more powerful and easier to write. Tools like Grunt or Gulp can automate the process of compiling your Sass files into regular CSS.
Integrating custom JavaScript
JavaScript can add interactivity and dynamic features to your WordPress theme. To include custom JavaScript, first create a js
directory in your theme folder and add your JavaScript files there. You can then enqueue these scripts in your functions.php
file using the wp_enqueue_script()
function.
For example, to add a custom script called scripts.js
, you would add the following code to your functions.php
file:
function my_theme_scripts() {
wp_enqueue_script('my-custom-script', get_template_directory_uri() . '/js/scripts.js', array('jquery'), '1.0.0', true);
}
add_action('wp_enqueue_scripts', 'my_theme_scripts');
This code ensures that your custom script is properly included in your theme. You can also enqueue third-party scripts, such as libraries and plugins, in the same way. Make sure to specify dependencies like jQuery to avoid conflicts and ensure proper loading order.
Additionally, consider using JavaScript frameworks or libraries, such as React or Vue.js, for more complex interactivity. These tools can help you create dynamic components and enhance the user experience on your site.
Using WordPress hooks and filters
WordPress hooks and filters are powerful tools that allow you to modify the default behavior of WordPress without altering core files. Hooks are functions that WordPress calls at specific points during execution, allowing you to insert custom code. Filters modify data before it is processed or displayed by WordPress.
Actions are a type of hook that lets you add custom code at specific points. For example, you can use the wp_head
action to insert code into the <head>
section of your HTML. Filters, on the other hand, modify data. For instance, the the_content
filter allows you to change the content of a post before it is displayed.
To use hooks and filters, add the relevant functions to your functions.php
file. For example, to add a custom function to the wp_footer
action, you would use the following code:
function my_custom_footer() {
echo '<p>Custom footer text</p>';
}
add_action('wp_footer', 'my_custom_footer');
Understanding and utilizing hooks and filters can greatly enhance the flexibility and functionality of your theme. They enable you to customize and extend WordPress in a modular and maintainable way.
Testing and debugging your theme
Once you’ve built your theme, it’s crucial to thoroughly test and debug it to ensure it works correctly across different browsers and devices. Start by using the WordPress Theme Unit Test Data to populate your site with sample content. This will help you identify any issues with your theme’s layout and functionality.
Use browser developer tools to inspect elements, check for errors, and test responsive design. Tools like Chrome DevTools allow you to simulate different devices and screen sizes, helping you ensure your theme looks good on all platforms. Validate your HTML and CSS using tools like the W3C Markup Validation Service and CSS Validator to catch any coding errors.
Finally, use debugging plugins like Query Monitor to identify performance issues and potential conflicts. This plugin provides detailed information about database queries, PHP errors, and scripts, making it easier to diagnose and fix problems. Regular testing and debugging are essential to delivering a high-quality, reliable WordPress theme.
Creating a custom WordPress theme involves several steps, from setting up your development environment to testing and debugging. By following these guidelines, you can build a unique, functional, and high-performing theme tailored to your website’s specific needs.